Table of Contents
Data Structures and Algorithms Interview Questions 2024
In today’s fast-paced technological world, businesses are generating massive amounts of data every day. To handle such an enormous volume of data, organizations rely on efficient data structures and algorithms. In computer science, data structures refer to the organization, storage, and management of data, whereas algorithms are a set of instructions that help solve a particular problem. By mastering data structures and algorithms, you can confidently tackle interview questions and showcase your ability to develop optimal solutions.
In this blog, we will explore the importance of data structures and algorithms in the tech industry, and discuss some common interview questions that you may encounter. So, whether you’re a seasoned software developer or a fresh graduate looking for your first job, read on to learn more about data structures and algorithms, and how to ace your next interview.
Sign up for the Skillvertex Data Structure course today and learn how to build efficient and effective programs with ease.
Data Structures and Algorithms Interview Questions
Here are 25 technical interview questions on data structures and algorithms:
1. What is a data structure?
Answer: A data structure is a way of organizing and storing data in a computer so that it can be used efficiently.
2. What are the different types of data structures?
Answer: The different types of data structures include arrays, linked lists, stacks, queues, trees, graphs, hash tables, and heaps.
3. What is the difference between an array and a linked list?
Answer: An array is a collection of elements of the same data type that are stored in contiguous memory locations. A linked list is a collection of elements, called nodes, that contain a value and a pointer to the next node.
4. What are a stack and a queue? How do they differ?
Answer: A stack is a data structure that follows the Last In First Out (LIFO) principle, meaning that the last element added to the stack is the first one to be removed. A queue is a data structure that follows the First In First Out (FIFO) principle, meaning that the first element added to the queue is the first one to be removed.
5. What is a binary tree? Can it be used for searching and sorting data?
Answer: A binary tree is a tree data structure in which each node has at most two children. Yes, a binary tree can be used for searching and sorting data.
6. What is a hash table? How does it work?
Answer: A hash table is a data structure that uses a hash function to map keys to values. The hash function takes the key as input and returns the index of the array where the value is stored.
Interested in learning more about data structures and algorithms? The Skillvertex Data Structure course offers a comprehensive introduction to these essential programming concepts. Sign up now and start building better programs.
7. What is the time complexity of different data structures like arrays, linked lists, trees, and graphs?
Answer: The time complexity of different data structures varies depending on the operation performed. For example, arrays have a constant time complexity for accessing elements, while linked lists have a linear time complexity.
8. What are the different types of algorithms?
Answer: The different types of algorithms include searching, sorting, dynamic programming, and greedy algorithms.
9. What is time complexity and space complexity? How do you calculate them?
Answer: Time complexity is the amount of time it takes for an algorithm to run as a function of the size of the input. Space complexity is the amount of memory used by an algorithm as a function of the size of the input. They are usually denoted by the Big O notation. For example, an algorithm with a time complexity of O(n) means that its running time increases linearly with the size of the input.
10. What is a sorting algorithm? Can you explain bubble sort, merge sort, and quicksort?
Answer: A sorting algorithm is an algorithm that puts elements in a specific order. Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Merge sort is a divide-and-conquer algorithm that divides the list into smaller sublists, sorts them, and then merges them back together. Quicksort is also a divide-and-conquer algorithm that picks an element as a pivot and partitions the array around it.
11. Explain the difference between a stack and a queue data structure.
Answer: A stack is a last-in, first-out (LIFO) data structure, whereas a queue is a first-in, first-out (FIFO) data structure.
12. What is the time complexity of inserting an element into a binary search tree?
Answer: The time complexity of inserting an element into a binary search tree is O(log n) in the average case and O(n) in the worst case.
13. What is the difference between a linked list and an array?
Answer: A linked list is a dynamic data structure where each element (node) stores a pointer to the next node in the list, whereas an array is a static data structure that stores a collection of elements of the same type in contiguous memory locations.
14. What is the difference between a depth-first search (DFS) and a breadth-first search (BFS) algorithm?
Answer: DFS explores as far as possible along each branch before backtracking, whereas BFS explores all the neighboring nodes at the current depth before moving on to the next level.
15. Explain the concept of dynamic programming.
Answer: Dynamic programming solves complex problems by breaking them down into smaller subproblems and solving each subproblem only once, storing the solution in a table to avoid redundant computations.
16. What is the time complexity of a linear search algorithm?
Answer: The time complexity of a linear search algorithm is O(n), where n is the size of the input array.
17. What is the difference between a hash table and a binary search tree?
Answer: A hash table is a data structure that uses a hash function to map keys to indices in an array, whereas a binary search tree is a data structure that stores key-value pairs in a tree-like structure where each node has at most two children.
18. Explain the concept of memorization.
Answer: Memorization is a technique for optimizing recursive algorithms by storing the results of expensive function calls and returning the cached result when the same inputs occur again.
19. What is the time complexity of a bubble sort algorithm?
Answer: The time complexity of a bubble sort algorithm is O(n^2), where n is the size of the input array.
20. What is the difference between a max heap and a min heap?
Answer: A max heap is a binary tree where each node has a value greater than or equal to its children, whereas a min heap is a binary tree where each node has a value less than or equal to its children.
21. Given a list of integers, write a function to return the second largest element.
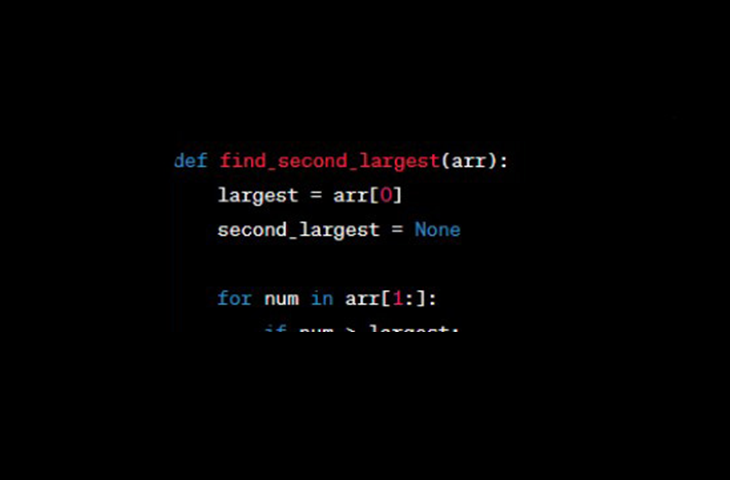
22. Write a function to check if a given string is a palindrome.
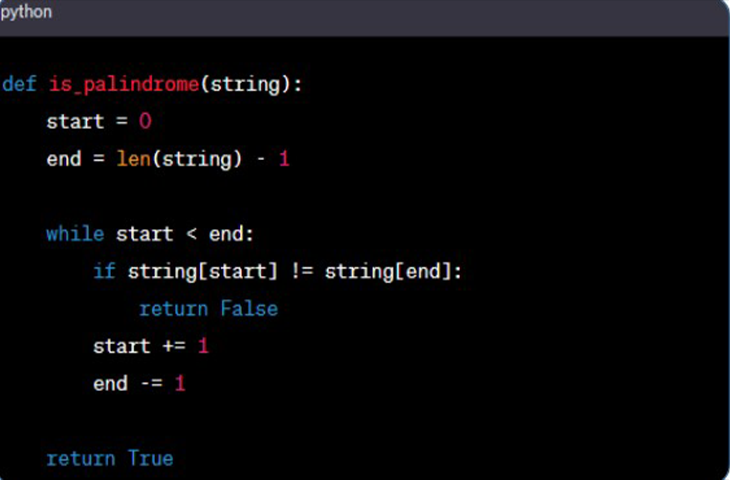
23. Given two sorted arrays, write a function to merge them into a single sorted array.
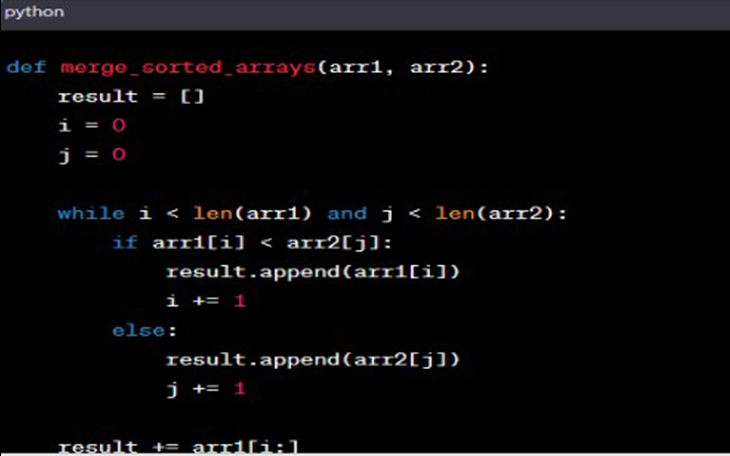
24. Write a function to find the shortest path between two nodes in a graph.
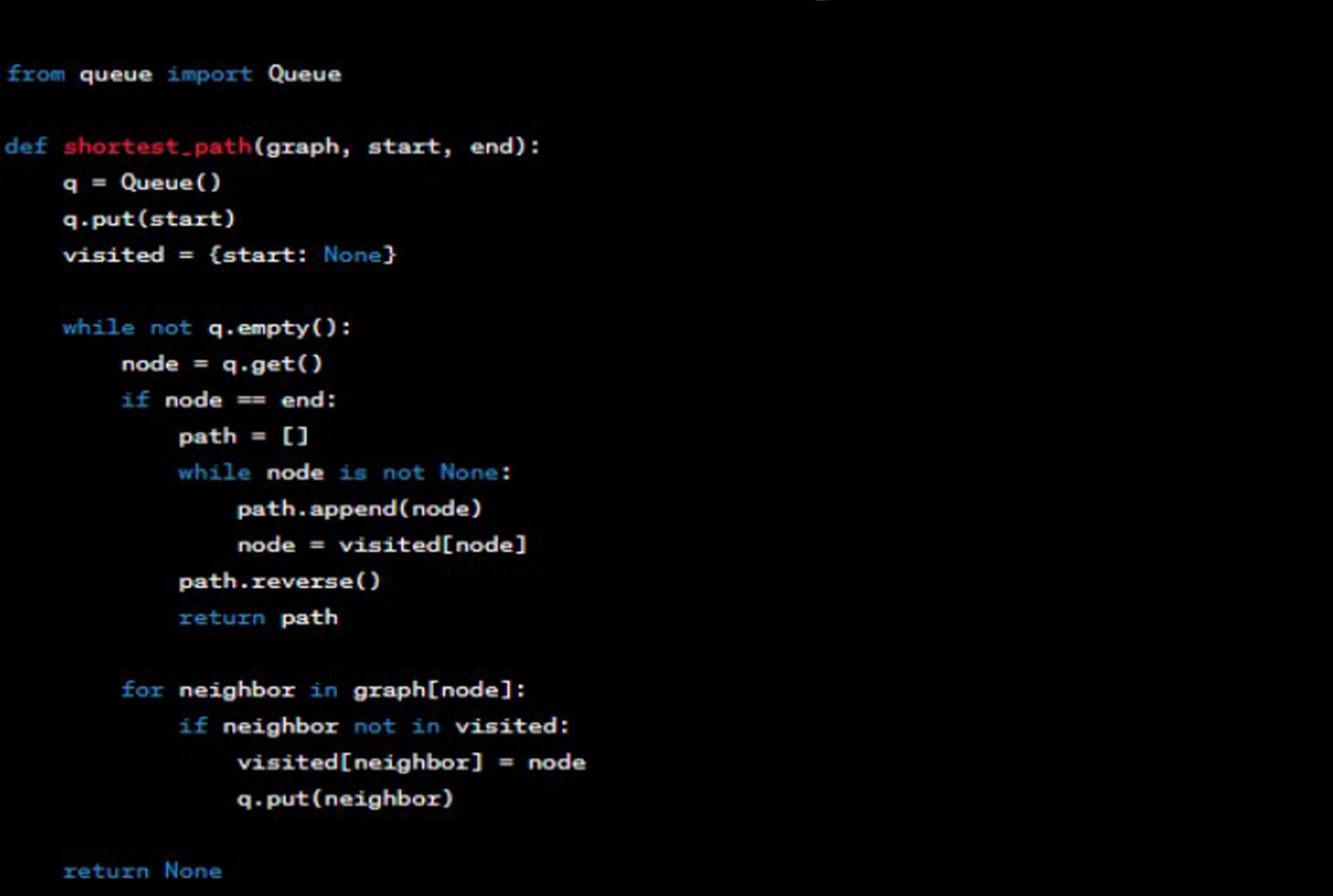
25. Implement a binary search algorithm to search for a specific element in a sorted array.
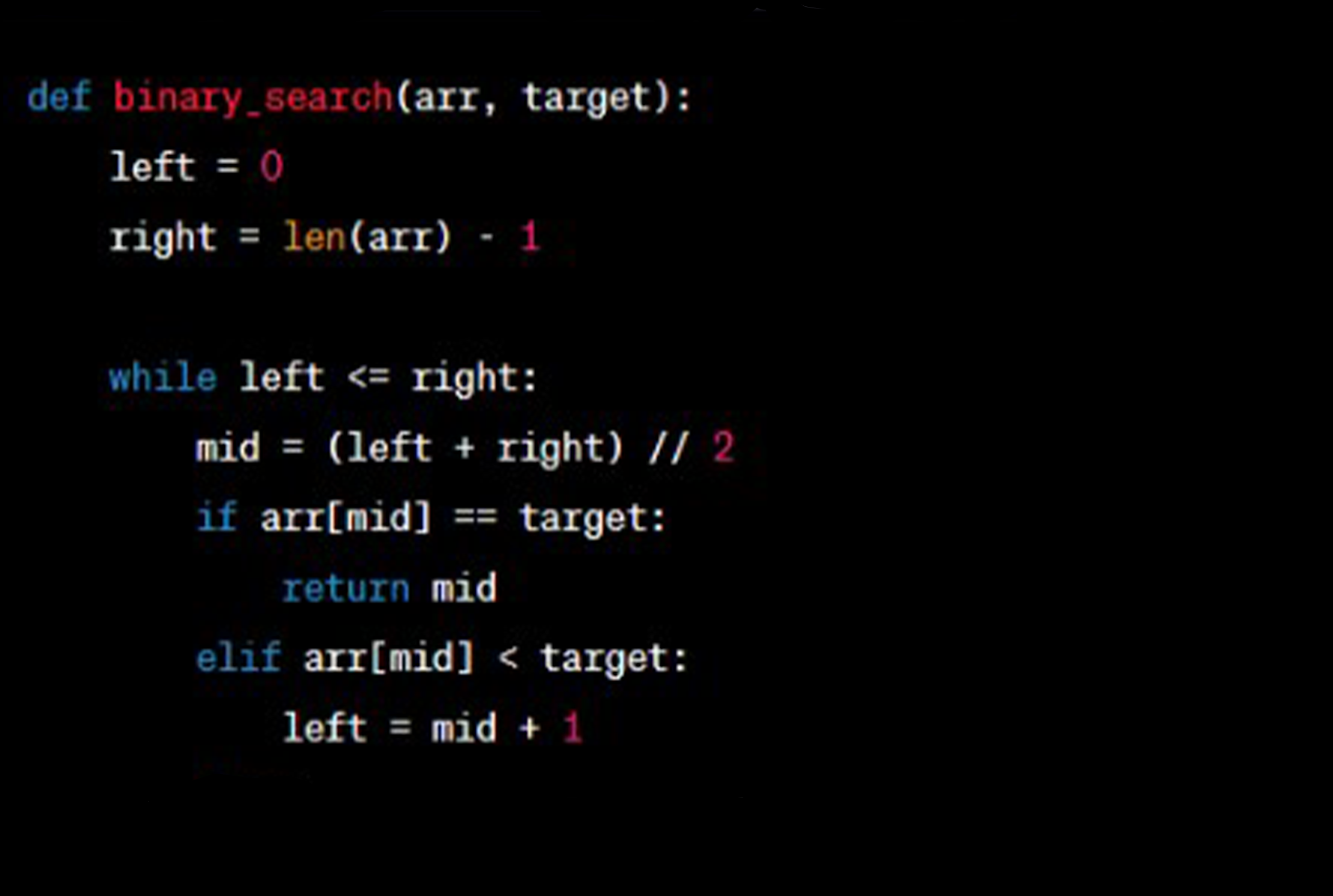
For each question, the interviewer may ask follow-up questions to clarify your approach and ask you to explain the time and space complexity of your solution. Additionally, they may ask you to optimize your solution or handle edge cases.
Whether you’re a beginner or an experienced programmer, the Skillvertex Data Structure course has something to offer. With industry experts & real-world applications, you’ll gain the skills you need to succeed in any programming role. Enroll today and start your journey to becoming a master programmer.
Data Structures and Algorithms Interview Questions PDF
we will shortly update the PDF version of Data Structures and Algorithms Interview Questions here.