What are Data structures, Data structure algorithms, and their types?
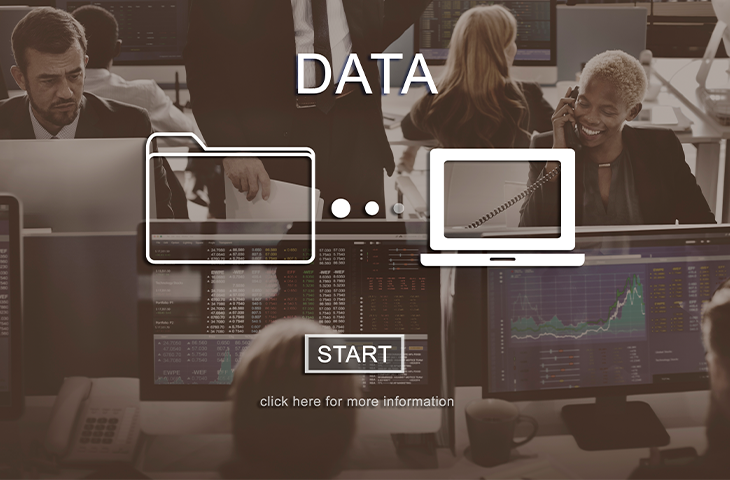
Algorithms and data structures are the foundation of effective programming. They serve as the foundation of every software system, allowing programmers to manipulate data in a systematic and effective way. Understanding and using data structures and algorithms is essential for developing reliable and scalable applications in the Java programming language.
Effective data management, storage, and retrieval are made possible by data structures. Every type of data structure, from straightforward arrays and linked lists to more intricate ones like trees, graphs, and hash tables, has its own special qualities and benefits. Developers may optimize operations like searching, sorting, insertion, and deletion by choosing the right data structure, which improves performance and reduces time complexity.
What are Data Structures?
Data structures serve as a means of organizing and storing data. It is a method of setting up data on a computer to make it easily accessible and up-to-date.
The best data format for your project should be chosen based on your requirements and project. For instance, you can use the Array data structure if you want to keep data in memory in a sequential manner.
Types of Data Structure
Basically, data structures are divided into two categories:
- Linear data structure
- Non-linear data structure
Linear data structures
The elements are placed sequentially, one after the other, in linear data structures. The elements are simple to use because they are set up in a specific order.
However, because of operational difficulties, linear data structures might not be the ideal option when program complexity rises.
1. Array Data Structure
The elements in memory are organized in a continuous memory array. An array’s items are all of the same type. Additionally, the programming language affects the kinds of elements that can be stored as arrays.
2. Stack Data Structure
The LIFO technique is used to store elements in stack data structures. In other words, the last element in a stack will be taken out first. It operates similarly to a stack of plates, where the final plate left on the pile is taken out first.
3. Queue Data Structure
Unlike stacks, the queue data structure works on the FIFO principle, where the first element stored in the queue will be removed first.
It works just like a queue of people at the ticket counter, where the first person in the queue will get the ticket first.
4. Linked List Data Structure
Data components in a linked list data structure are linked together by a number of nodes. Additionally, each node has an address for the node behind it as well as data elements.
Non-linear data structures
Non-linear data structures differ from linear data structures in that their elements are not in any particular order. Instead, they are arranged hierarchically, with each piece related to another on some level.
Graph- and tree-based data structures are subsets of non-linear data structures.
1. Graph Data Structure
Each node is referred to as a vertex in a graph data structure, and each vertex is connected to other vertices through edges.
2. Trees Data Structure
A tree is a collection of vertices and edges, much like a graph. However, there can only be one edge between any two vertices in a tree data structure.
What are algorithms?
An algorithm is a process with well-defined steps for solving a specific problem. Or, to put it another way, an algorithm is a limited set of rules or instructions that are used to carry out a certain task that has been predetermined. A flowchart or pseudocode can be used to illustrate the answer (logic), which is all that is needed to solve a problem and not the entire program or code.
1. Sorting Algorithms
Sorting algorithms are detailed processes for moving data around in lists and arrays. An array may need to be sorted, for instance, in numerical or lexical order. Searching algorithms, for example, are made more effective by sorting algorithms.
Three basic sorting algorithms are insertion sort, merge sort, and rapid sort.
Insertion Sort
Insertion sorting is a useful method for completing the task of sorting tiny data sets that are almost sorted. An array is split into sorted and unsorted portions using this procedure. Once all of the items are sorted, it picks one from the unsorted section and moves it to the sorted part.
Merge Sort
Linked lists are best organized using a merge sort. A list is split in half until it can no longer be divided. After that, it compares and combines the pieces in the same way they were split apart.
QuickSort
Quicksort is beneficial for large data sets. It divides an array into two subarrays based on the pivot, a designated data element. Elements in the first subarray have values lower than the pivot. Elements in the second subarray have values higher than the pivot. The algorithm locates the pivot in each subarray until there is just one element in each subarray.
2. Searching Algorithms
Specific elements within data structures are located and retrieved using search methods. Binary search and linear search are two prime examples.
Linear Search
A sequential searching strategy for both sorted and unsorted data types is linear search. It moves through lists and arrays one element at a time, sequentially.
Consider a list of unsorted entries with the values “1” through “25.” The values would be explored in the order they are stored in a linear search for the value “5”.
Binary Search
An interval searching algorithm is binary search. Divided into many intervals, interval searches only travel over the desired interval. Because they divide the search space, they are more effective than sequential searches.
A sorted list can be efficiently searched for elements using binary search. It then moves across the anticipated interval after comparing the search value to the data structure’s middle element. Think about a sorted list of elements that ranges from “1” to “25.” If you were to perform a binary search for the value “5”, it would be compared to the middle element, “13.” Since “5” is smaller than “13,” the algorithm would look for the value in the lower half of the interval.
3. Graph Traversal Algorithms
In order to search nodes in graphs and trees, computer scientists employ graph traversal techniques. In contrast to linear data structures used in computer science, graphs require many searches in order to locate and retrieve data.
The breadth-first search and the depth-first search are two graph traversal techniques. They aid computer experts in resolving the most prevalent graph and tree-related issues.
Breadth-First Search
The shortest route between two nodes is traversed using breadth-first search (BFS). It investigates nodes in decreasing order of distance, starting at the tree’s base.
BFS would go from left to right in the following order when searching nodes in a tree with two tiers of nodes:
- Tree root
- Nodes in the first level
- Nodes in the second level
Depth-First Search
From top to bottom, depth-first search (DFS) scans graphs. It travels as far as it can down one branch before turning around and moving on to the next.
Three methods exist for implementing DFS:
Starting from the tree’s root, the preorder traversal first travels through the left subtree before moving on to the right subtree.
In-order Starting at the left subtree, the traversal proceeds to the tree root, then to the right subtree.
Post-order Traversal: The tree root is reached after traversing the left subtree and the right subtree first.
4. Dynamic Programming Algorithms
Algorithms for dynamic programming are the next on the list of several sorts of algorithms. Dynamic programming is a technique for both computer programming and mathematical optimization. The approach was created by Richard Bellman in the 1950s and has found use in a wide range of disciplines, including economics and aerospace engineering.
It refers to the process of recursively decomposing a complex problem into smaller, simpler problems in order to make it simpler. Even though some decision problems can’t be broken down in this way, recursive breakdowns of decisions that span several points in time is common. Similar to this, an issue is considered to have an optimal solution in computer science if it can be solved by first decomposing it into smaller problems and then recursively finding the best answers to those smaller problems.
The following computer problems can be solved using a dynamic programming approach −
- Fibonacci number series
- Knapsack problem
- Tower of Hanoi
- All pair shortest path by Floyd-Warshall
- Shortest path by Dijkstra
- Project scheduling
5. Greedy Algorithms
Any algorithm that makes the locally optimal decision at each stage when addressing a problem is said to be greedy. In many cases, a greedy method does not always result in the best answer; rather, a greedy heuristic may create locally optimal solutions that, in a reasonable length of time, approach a globally optimal solution.
For instance, the following heuristic is a greedy solution to the traveling salesman problem, which has a high computing complexity: “At each step of the journey, visit the nearest unvisited city.” This heuristic terminates in a fair number of steps, but it does not aim to discover the best solution; ordinarily, an optimal solution to a problem of this complexity necessitates an unreasonable number of steps. In mathematical optimization, greedy algorithms give constant-factor approximations to problems with submodular structures and solve combinatorial problems with matroid-like qualities in the best possible way.
Most networking algorithms use a greedy approach. Here is a list of a few of them:
- Traveling Salesman Problem
- Prim’s Minimal Spanning Tree Algorithm
- Kruskal’s Minimum Spanning Tree Algorithm
- Dijkstra’s Minimum Spanning Tree Algorithm
- Graph: Map Coloring
- Graph: Vertex Cover
In conclusion, data structures and algorithms are key ideas in computer science that make it possible to organize, manipulate, and solve problems with data effectively. From simple arrays to intricate structures like trees and graphs, data structures offer a methodical means of storing and accessing data. Algorithms, on the other hand, are detailed instructions or processes created to address particular computational issues by utilizing the strengths of data structures. They cover a wide range of algorithms, each with a specific function, such as searching, sorting, graph traversal, and dynamic programming. Programmers may efficiently and elegantly solve complicated problems by optimizing their code, using various data structures and techniques, and enhancing performance. For the purpose of creating scalable and reliable software solutions, mastery of these ideas is essential.