Data Structure algorithm interview Question
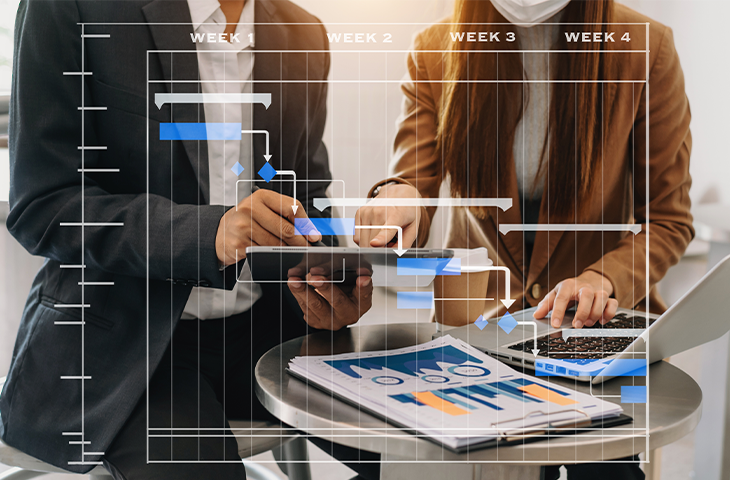
Data structures and algorithms are fundamental ideas in computer science that are essential to effectively resolving challenging issues. A data structure is a method for organizing and storing data in a computer’s memory to facilitate effective information manipulation and retrieval. An algorithm, on the other hand, is a step-by-step process or a collection of guidelines for resolving a specific problem. Data structures and algorithms work as the foundation for creating software systems that are effective and optimized.
On the other side, algorithms are the set of guidelines or rules that specify how data is altered or processed. They include a broad range of methods, including graph traversal, search, sorting, dynamic programming, and divide-and-conquer. In order to be as simple as possible, an algorithm’s time complexity and memory requirements must be kept to a minimum. Programmers can enhance the functionality of their programmes by algorithmic analysis and design, making it possible for them to manage huge datasets, challenging computations, and real-time processing.
11 interview Questions
- How would you use a stack to reverse a string?
These steps can be used to reverse a string using a stack:
Make a blank stack.
Go through each character in the string repeatedly.
Each character should be pushed onto the stack.
Pop each character from the stack and add it to a new string once you have reached the end of the previous string.
The original string’s reversed form will be used in the new string.
Here’s an example implementation in Python:
Example usage:
# Output: “!dlroW ,olleH”
In this example, the string “Hello, World!” is reversed using a stack, resulting in the output “!dlroW ,olleH”.
2. How does a priority queue work? Provide an example.
An abstract data type called a priority queue makes it possible to insert and remove components quickly according to their priority. Each item in the priority queue has a priority value assigned to it, and items with higher priorities are removed from the queue before items with lower priorities. A heap data structure is frequently used in the implementation of priority queues.
Here is a brief description of how a priority queue operates:
The priority queue receives the elements according to their distinct priorities.
Always at the head of the queue is the item with the highest priority.
The element with the following greatest priority moves to the front when an element is dequeued.
According to the implementation approach chosen (for example, first-in-first-out), elements with the same priority are handled differently.
Here is an illustration of how to create a priority queue in Python using the native heapq module:
python
Copy code
import heapq
class PriorityQueue:
def __init__(self):
self._queue = []
self._index = 0
def is_empty(self):
return len(self._queue) == 0
def enqueue(self, item, priority):
heapq.heappush(self._queue, (priority, self._index, item))
self._index += 1
def dequeue(self):
if self.is_empty():
raise IndexError(“Priority queue is empty”)
return heapq.heappop(self._queue)[-1]
Example usage:
Output:
arduino
Copy code
Task 2
Task 3
Task 1
In this example, we enqueue three tasks into the priority queue with their respective priorities. The task with the highest priority (lowest value) is dequeued first, resulting in the output order “Task 2”, “Task 3”, “Task 1”.
3. Explain the concept of dynamic programming.
Using a bottom-up approach, dynamic programming is a strategy for addressing problems that divides larger, more complex problems into smaller, overlapping subproblems. By saving and reusing answers to subproblems and cutting out unnecessary computations, it reduces the time complexity. It entails establishing basic cases, recognising the problem, developing a table to store solutions, populating the table, and building the ultimate solution. Dynamic programming provides effective solutions by minimising superfluous calculations for problems with optimal substructure and overlapping subproblems.
4. How would you implement a graph data structure?
Different methods can be used to implement a graph data structure. An adjacency list or adjacency matrix are two methods that are frequently used to represent a graph.
Adjacency List: In this method, we represent the graph’s vertices using an array or a hash map.
A list or an array that contains its neighboring vertices is linked to each vertex in the array/hash map.
Adjacency lists make it feasible to efficiently represent sparse graphs, which are those with a much lower number of edges than the total number of edges that can be present.
Here is a Python implementation example:
class Graph:
def __init__(self):
self.graph = {}
def add_vertex(self, vertex):
if vertex not in self.graph:
self.graph[vertex] = []
def add_edge(self, source, destination):
if source in self.graph and destination in self.graph:
self.graph[source].append(destination)
self.graph[destination].append(source) # For an undirected graph
def get_neighbors(self, vertex):
if vertex in self.graph:
return self.graph[vertex]
return []
# Example usage:
# Output: [‘A’, ‘C’]
Adjacency Matrix:
In this approach, we use a 2D matrix to represent the edges between vertices.
The rows and columns of the matrix correspond to the vertices, and the values in the matrix indicate the presence or absence of an edge between two vertices.
An adjacency matrix allows for efficient representation of dense graphs (where the number of edges is close to the maximum possible edges).
Here’s an example implementation in Python:
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.graph = [[0] * num_vertices for _ in range(num_vertices)]
def add_edge(self, source, destination):
if 0 <= source < self.num_vertices and 0 <= destination < self.num_vertices:
self.graph[source][destination] = 1
self.graph[destination][source] = 1 # For an undirected graph
def get_neighbors(self, vertex):
if 0 <= vertex < self.num_vertices:
neighbors = []
for i in range(self.num_vertices):
if self.graph[vertex][i] == 1:
neighbors.append(i)
return neighbors
return []
# Example usage:
# Output: [0, 2]
These are two common approaches for implementing a graph data structure. The choice between an adjacency list and an adjacency matrix depends on the characteristics of the graph and the specific requirements of the problem at hand.
5. How would you check if a binary tree is a binary search tree?
You can use an inorder traversal and check the elements’ order to see if a binary tree is a binary search tree (BST). The inorder traversal of a binary search tree results in a sorted series of entries.
The following is a general approach to determine whether a binary tree is a binary search tree:
run the binary tree through an inorder traverse.
Compare each element with the one before it during the traversal.
The tree cannot be a valid BST if any element is smaller than or equal to its forerunner.
The tree is a valid BST if the traverse is completed with no violations.
Here is a Python implementation example:
class TreeNode:
def __init__(self, value):
self.val = value
self.left = None
self.right = None
def is_bst(root):
stack = []
prev = None # To store the previous element during traversal
while root or stack:
while root:
stack.append(root)
root = root.left
root = stack.pop()
if prev and root.val <= prev.val:
return False
prev = root
root = root.right
return True
# Example usage:
# Check if the tree is a binary search tree
if is_bst(root):
print(“The binary tree is a binary search tree.”)
else:
print(“The binary tree is not a binary search tree.”)
In this example, the binary tree is constructed with values that satisfy the properties of a BST. The algorithm performs an inorder traversal and checks if the elements are in ascending order. Since the traversal completes without any violations, it confirms that the tree is a valid binary search tree.
Please note that this algorithm assumes that the binary tree does not contain duplicate values. If the tree allows duplicate values, additional rules or constraints need to be considered to determine if it is a binary search tree.
6. Compare and contrast a stack and a queue.
A stack and a queue are both abstract data types used to store and retrieve elements, but they differ in their fundamental principles and operations:
Stack:
Principle: The stack follows the Last-In-First-Out (LIFO) principle.
Operations:
Push: Adds an element to the top of the stack.
Pop: Removes and returns the topmost element from the stack.
Peek/Top: Returns the value of the topmost element without removing it.
Visualization: Imagine a stack of plates. You can only add or remove plates from the top.
Example usage: Function call stack, undo/redo operations.
Queue:
Principle: The queue follows the First-In-First-Out (FIFO) principle.
Operations:
Enqueue: Adds an element to the back (or end) of the queue.
Dequeue: Removes and returns the frontmost (or first) element from the queue.
Front: Returns the value of the frontmost element without removing it.
Rear/Back: Returns the value of the rearmost element without removing it.
Visualization: Think of a queue of people waiting in line. New people join at the rear, and the person at the front is served and leaves.
Example usage: Task scheduling, breadth-first search.
Comparison:
Ordering: Stack follows LIFO, while queue follows FIFO.
Insertion and Deletion: Stacks allow for efficient insertion and deletion at one end (top), while queues allow for efficient insertion at one end (rear) and deletion at the other end (front).
Access: Stacks only allow access to the topmost element, while queues allow access to both the front and rear elements.
Usage: Stacks are useful for tracking function calls, managing recursive algorithms, and maintaining a history of actions. Queues are suitable for handling tasks in a sequential manner, managing resources, and breadth-first traversal of graphs.
Data Structure: Stacks can be implemented using arrays or linked lists. Queues can also be implemented using arrays or linked lists.
In summary, while both stacks and queues are used to store and retrieve elements, their core principles (LIFO vs. FIFO) and associated operations (push/pop vs. enqueue/dequeue) differentiate their behaviors and applications.
7. Compare and contrast a min-heap and a max-heap.
A min-heap and a max-heap are both binary trees that satisfy the heap property, but they differ in how that property is defined:
Min-Heap:
Heap Property: In a min-heap, for any given node, the value of that node is smaller than or equal to the values of its children.
Root Element: The root element of a min-heap is the minimum element in the heap.
Operations:
Insertion: New elements are inserted at the next available position in the tree and then “bubbled up” if necessary to maintain the heap property.
Deletion: The minimum element (root) is removed from the heap, and the last element in the tree is moved to the root position. Then, the element is “bubbled down” if necessary to restore the heap property.
Use Cases: Min-heaps are commonly used for priority queues, where the element with the smallest priority value should be dequeued first.
Max-Heap:
Heap Property: In a max-heap, for any given node, the value of that node is greater than or equal to the values of its children.
Root Element: The root element of a max-heap is the maximum element in the heap.
Operations:
Insertion: New elements are inserted at the next available position in the tree and then “bubbled up” if necessary to maintain the heap property.
Deletion: The maximum element (root) is removed from the heap, and the last element in the tree is moved to the root position. Then, the element is “bubbled down” if necessary to restore the heap property.
Use Cases: Max-heaps are often used for priority queues, where the element with the largest priority value should be dequeued first. They can also be used in algorithms such as heap sort.
Comparison:
Ordering: In a min-heap, the minimum element is at the root, while in a max-heap, the maximum element is at the root.
Heap Property: In a min-heap, the value of any node is smaller than or equal to the values of its children, while in a max-heap, the value of any node is greater than or equal to the values of its children.
Insertion and Deletion: Both min-heaps and max-heaps use similar algorithms for insertion and deletion but with different comparisons based on the heap property.
Use Cases: Min-heaps and max-heaps have similar use cases, such as priority queues, but their respective heap properties determine whether the smallest or largest element is prioritized.
In summary, min-heaps and max-heaps differ in their heap property, the ordering of elements, and the way elements are compared during insertion and deletion. They are both efficient data structures for maintaining a partially ordered binary tree, and their specific properties make them suitable for different applications depending on whether the smallest or largest element is of interest.
8. Describe the process of finding the first non-repeating character in a string.
The process of finding the first non-repeating character in a string involves iterating through the string and keeping track of the frequency of each character. Here’s a step-by-step approach to solve this problem:
Create an empty hash map or dictionary to store the frequency of each character in the string.
Iterate through the string and update the frequency count for each character.
After the iteration, iterate through the string again and check the frequency count for each character.
Return the first character that has a frequency count of 1.
Here’s an example implementation in Python:
# Output: ‘c’
In this example, the string “abracadabra” is passed to the first_non_repeating_char function. The function counts the frequency of each character using a hash map. It then iterates through the string again and returns the first character that has a frequency count of 1, which is ‘c’ in this case.
The time complexity of this algorithm is O(n), where n is the length of the input string, as we iterate through the string twice. The space complexity is O(k), where k is the number of distinct characters in the string, as we store the frequency count in a hash map.
9. How would you check if a linked list is a palindrome?
To check if a linked list is a palindrome, you can utilize the concept of a two-pointer approach. Here’s the step-by-step process to solve this problem:
Find the middle node of the linked list using the slow and fast pointer technique. The slow pointer moves one step at a time, while the fast pointer moves two steps at a time. When the fast pointer reaches the end of the list, the slow pointer will be at the middle node.
Reverse the second half of the linked list starting from the node after the middle node.
Compare the values of the first half of the original linked list (from the start to the middle) with the reversed second half of the list.
If all the values match, the linked list is a palindrome. Otherwise, it is not.
Here’s an example implementation in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def is_palindrome(head):
# Find the middle node using the slow and fast pointer technique
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
# Reverse the second half of the linked list
prev = None
while slow:
next_node = slow.next
slow.next = prev
prev = slow
slow = next_node
# Compare the first half and the reversed second half
first_half = head
second_half = prev
while second_half:
if first_half.val != second_half.val:
return False
first_half = first_half.next
second_half = second_half.next
return True
# Example usage:
if is_palindrome(head):
print(“The linked list is a palindrome.”)
else:
print(“The linked list is not a palindrome.”)
In this example, a linked list with values 1, 2, 3, 2, 1 is created. The is_palindrome function uses the two-pointer approach to find the middle node, reverse the second half, and compare the first half with the reversed second half. Since all the values match, the function outputs that the linked list is a palindrome.
The time complexity of this algorithm is O(n), where n is the number of nodes in the linked list, as we iterate through the list twice. The space complexity is O(1) as we perform the operations in-place without using any additional data structures that grow with the size of the input.
10. What is a circular linked list? How would you detect if a linked list is circular?
A circular linked list is a type of linked list where the last node in the list points back to the first node, forming a loop or cycle. In other words, the “next” pointer of the last node points to a node earlier in the list, rather than being set to null as in a regular singly linked list.
To detect if a linked list is circular, you can use the concept of a slow and fast pointer. Here’s the step-by-step process:
Initialize two pointers, slow and fast, to the head of the linked list.
Move the slow pointer one step at a time and the fast pointer two steps at a time.
If the linked list is not circular, the fast pointer will reach the end (null) before the slow pointer.
If the linked list is circular, the fast pointer will eventually “catch up” to the slow pointer and they will meet at some node.
If the fast pointer and slow pointer meet, it indicates that the linked list is circular.
Here’s an example implementation in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def is_circular(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while fast and fast.next:
if slow == fast:
return True
slow = slow.next
fast = fast.next.next
return False
# Example usage:
if is_circular(head):
print(“The linked list is circular.”)
else:
print(“The linked list is not circular.”)
In this example, a circular linked list is created with values 1, 2, 3, and 4. The last node points back to the second node, creating a loop. The is_circular function uses the slow and fast pointer approaches to detect circularity. Since the fast pointer eventually catches up to the slow pointer, indicating that they have met, the function outputs that the linked list is circular.
The time complexity of this algorithm is O(n), where n is the number of nodes in the linked list. The space complexity is O(1) as we only use a constant amount of additional memory for the two pointers.
11. Describe the concept of recursion and provide an example.
Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, similar subproblems. In recursive algorithms, a base case is defined to stop the recursion and return a result, while the recursive case invokes the function on a smaller or simpler input to make progress towards the base case.
The key components of a recursive function are:
Base Case: The condition that defines the simplest form of the problem, where no further recursive calls are needed. It provides the stopping condition for the recursion.
Recursive Case: The condition that defines the problem in terms of smaller or simpler subproblems. It involves making one or more recursive calls with modified input parameters to eventually reach the base case.
Recursion is often used to solve problems that exhibit self-replicating or self-referencing structures, such as tree traversal, searching, sorting, and more.
Here’s an example to demonstrate recursion in action:
def factorial(n):
# Base case: factorial of 0 or 1 is 1
if n == 0 or n == 1:
return 1
# Recursive case: factorial of n is n multiplied by factorial of (n-1)
else:
return n * factorial(n-1)
# Example usage:
# Output: 120
In this example, the factorial function calculates the factorial of a number using recursion. When factorial(n) is called, it checks if n is 0 or 1 (the base case). If so, it returns 1. Otherwise, it makes a recursive call to factorial(n-1) (the recursive case) and multiplies the result by n. This recursive process continues until the base case is reached, at which point the results are accumulated and returned.
When factorial(5) is called, it recursively calculates 5 * factorial(4), 4 * factorial(3), 3 * factorial(2), 2 * factorial(1), and finally 1 * factorial(0). Since the base case is encountered with factorial(0) and factorial(1), the recursive calls return their results, and the multiplication chain is resolved to give the final result of 120.
It’s important to ensure that a recursive function has proper termination conditions (base case) and that the recursive calls lead towards the base case. Otherwise, it can result in infinite recursion and stack overflow errors.